1.1 Overview of Python
What is Python?
Python is a high-level, interpreted programming language known for its simplicity, readability, and versatility. Created by Guido van Rossum and first released in 1991, Python is designed to emphasize code readability with its clean and straightforward syntax, making it an ideal language for both beginners and experienced developers.
Python allows developers to write clear and logical code for small to large-scale projects and is widely used in areas such as web development, data analysis, machine learning, automation, and scientific computing. Its design philosophy is built around making programming as accessible and efficient as possible.
Key Features of Python:
- Interpreted Language
Python is an interpreted language, which means the code is executed line by line. You don't need to compile Python code before running it, which makes debugging and testing much easier. The Python interpreter processes the code at runtime and provides immediate feedback. - Cross-Platform
Python is cross-platform, which means it can run on various operating systems like Windows, macOS, and Linux. The same Python code will generally work on any of these systems without modification, making it highly portable. - Extensive Standard Library
One of Python's biggest strengths is its large standard library. Python comes with modules for a wide range of programming tasks, such as handling file I/O, interacting with operating system functions, working with data structures, and performing math operations. This eliminates the need for writing extensive code to implement common functionality. - Third-Party Libraries and Frameworks
In addition to the standard library, Python has a vast ecosystem of third-party libraries and frameworks available through the Python Package Index (PyPI). These libraries make it easier to implement specialized tasks such as web development (with Django, Flask), data science (with NumPy, Pandas), machine learning (with TensorFlow, Scikit-learn), and more. - Open Source and Community-Driven
Python is an open-source language, which means its source code is freely available to the public. Python has a large and active community of developers who contribute to its ongoing development and maintain a wide variety of open-source projects. This community support provides valuable resources, including documentation, tutorials, and troubleshooting help.
Dynamic Typing
In Python, you don't need to explicitly declare variable types. The type of a variable is dynamically inferred by the interpreter at runtime, depending on the value you assign to it.Example:
x = 10 # x is an integer
x = "Python" # Now, x is a string
Readability and Simplicity
Python's syntax is designed to be easy to read and understand. It relies on indentation to define code blocks, which reduces the need for explicit brackets and encourages clean coding practices. This makes Python an excellent language for beginners, as its code is often more intuitive than other languages like C++ or Java.Example of a simple Python code snippet:
# This is a comment in Python
print("Hello, World!")
Applications of Python
Python's versatility allows it to be used in a variety of domains:
- Web Development
Python provides powerful frameworks like Django and Flask for building web applications. These frameworks help developers create secure and scalable websites quickly. - Data Science and Machine Learning
Python is widely used in data science for data analysis, visualization, and machine learning. Libraries such as NumPy, Pandas, Matplotlib, and TensorFlow allow developers to analyze large datasets and build machine learning models. - Automation (Scripting)
Python is often used for automating repetitive tasks. From automating file management to web scraping, Python scripts can handle many tasks that would otherwise require manual intervention. - Scientific Computing
Python is popular in scientific computing and research. Libraries such as SciPy and SymPy are used for performing complex mathematical operations, simulations, and solving scientific problems. - Game Development
Python is used for game development with libraries like Pygame, which provides functionality for writing simple 2D games. - Artificial Intelligence (AI)
Python is at the forefront of AI and machine learning. Libraries like TensorFlow, Keras, and PyTorch enable developers to implement deep learning models for tasks like image recognition, natural language processing, and autonomous systems.
Advantages of Python
- Easy to Learn and Use
Python's simple syntax and semantics make it one of the easiest programming languages to learn. This is why Python is often the language of choice for beginners, yet powerful enough for advanced users. - Large Community and Support
Python has a vast user base and a robust online community that contributes to forums, open-source projects, and comprehensive documentation. This support makes it easy to find solutions to problems and learn from the experiences of other developers. - Extensible and Embeddable
Python can be extended using C or C++, allowing you to write performance-critical code in these lower-level languages. Additionally, Python can be embedded into larger applications as a scripting language. - Productivity and Speed
Python's high-level nature allows developers to write less code compared to languages like Java or C++. This leads to higher productivity and faster prototyping, making Python an excellent language for rapid application development.
Disadvantages of Python
- Performance
Since Python is an interpreted language, it tends to be slower compared to compiled languages like C++ or Java. However, for most use cases, the performance trade-off is acceptable, and there are ways to optimize Python code or write performance-critical parts in other languages. - Memory Usage
Python can consume more memory than other programming languages, particularly when handling large datasets. This can be mitigated by careful optimization and using memory-efficient data structures. - Mobile Development
Python is not commonly used for mobile application development. While libraries like Kivy exist for building mobile apps, other languages like Java (for Android) and Swift (for iOS) are more commonly used.
Conclusion
Python is a versatile, powerful, and easy-to-learn programming language, making it a great choice for a wide range of applications, from web development and automation to data science and machine learning. Its simple syntax and rich ecosystem of libraries allow developers to quickly build robust applications. Whether you're a beginner or an experienced developer, Python offers the tools and flexibility to tackle any programming challenge.
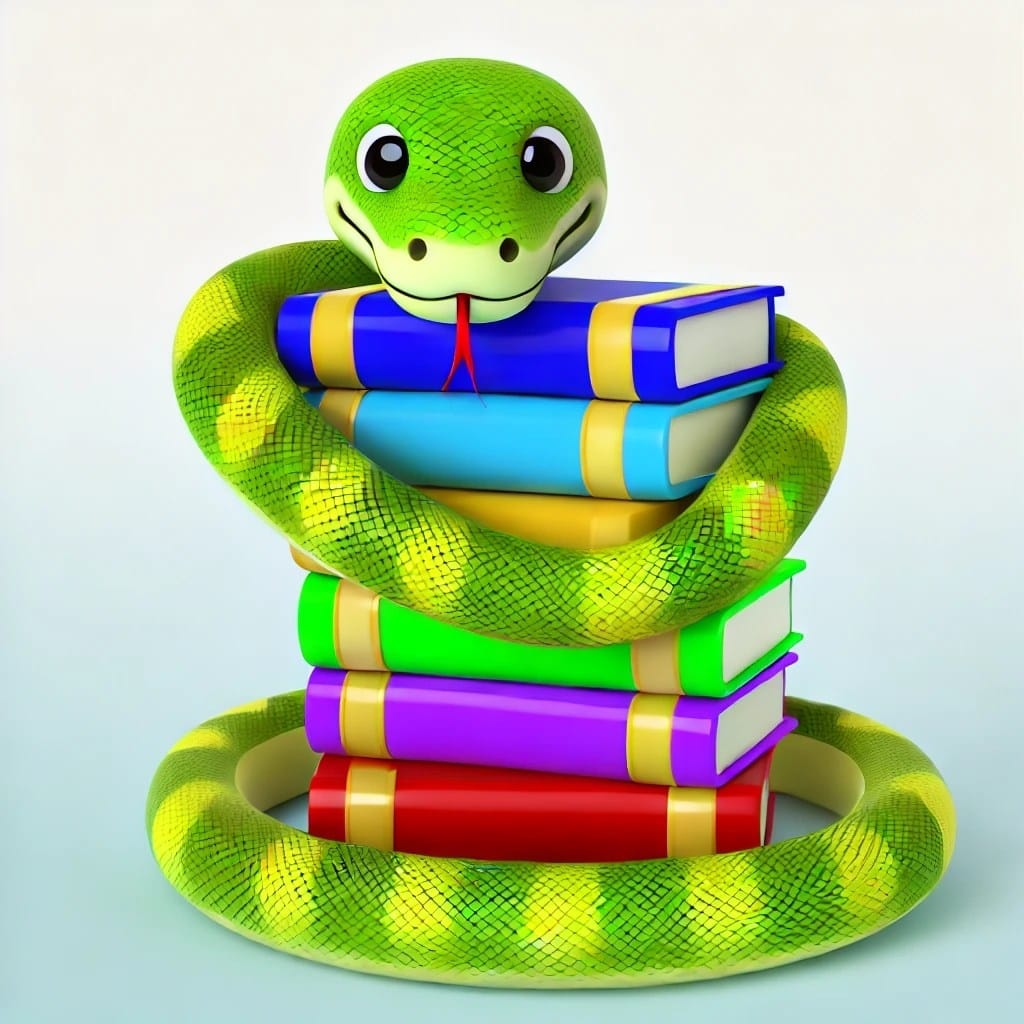